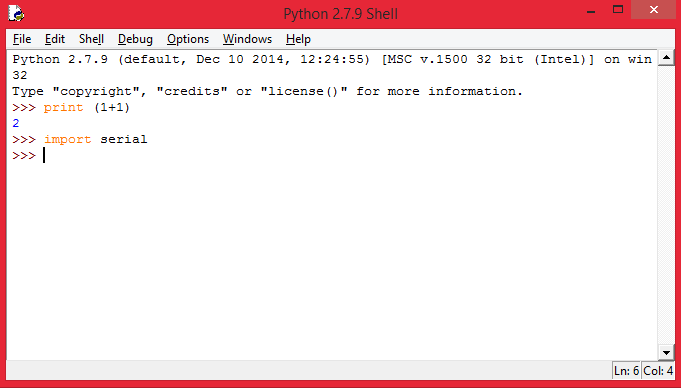
For anyone who would like to interface with their car, here is a guide to do so.
Python Serial Timeout Example Documentation
The following are 7 code examples for showing how to use serial.PARITYODD. These examples are extracted from open source projects. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example. You may check out the related API usage on the sidebar. Welcome to pySerial’s documentation¶. This module encapsulates the access for the serial port. It provides backends for Python running on Windows, OSX, Linux, BSD (possibly any POSIX compliant system) and IronPython. The module named “serial” automatically selects the appropriate backend.
For this you need a few things:
- A car supporting OBD2 (most cars since 1996 have this).
- A laptop/computer running Python 2.7.3
- An OBD connector (available online – I have an ELM327 bluetooth one which cost under €10)
So now that you have those, lets get started:
Connecting to the OBD2 connector:
Whether you are using a Bluetooth or USB OBD2 connection, it is a serial connection. To talk to it through python you can use pyserial.
You can list all serial ports on OSX or Linux by typing the following in the terminal:
ls /dev/tty*
(“ls” above is short for “list the following with file name” and the “/dev/tty” is the start of the file path of any serial port).
Take note of the serial device you are using – you will need it below.
- Download pyserial and install on your machine.
- Open the terminal (command prompt on Windows).
- Type
python
to start Python. - Type
import serial
to make use of Pyserial - Now connect to the serial device. To do this you will need the name of the serial device you are using (see above). Type the following:
ser = serial.Serial('NAME_OF_SERIAL_DEVICE', 38400, timeout=1)
- Now you’ve connected to the OBD so you can start sending commands. For example, to measure speed type:
ser.write('01 0D r')
A full list of OBD commands can be obtained here. - The elm327 device returns values in HEX. To read the value you just requested in Python type
speed_hex = ser.readline().split(' ')
- Convert the HEX to decimal by using:
speed = float(int('0x'+speed_hex[3], 0 ))
- Finally output to the screen:
print 'Speed: ', speed, 'km/h'
Now you should see the speed of your vehicle appear on screen.
Python Readline Timeout
Any issues/questions shout below in the comments and I will try to help out!
My next project is to get all of this onto a Raspberry Pi so I can monitor the car’s ECU on the go.
Interfacing with a RS232 serial device is a common task when using Python in embedded applications. The easiest way to get python talking to serial ports is use the pyserial project found at http://pyserial.sourceforge.net/. This module works on most platforms and is straightforward to use (see examples on project web site). However, getting the read function in this module to operate in an optimal way takes a little study and thought. This article investigates how the pyserial module works, possible issues you might encounter, and how to optimize serial reads.
We start out with several goals as to how we want the application to behave in relation to the serial port:
- application must block while waiting for data.
- for performance reasons, we want to read decent size chunks of data at a time if possible. Python function calls are expensive, so performance will be best if we can read more than one byte at a time.
- We want any data received returned in a timely fashion.
A key parameter in the pyserial Serial class is the timeout parameter. This parameter is defined as:

The Serial class read function also accepts a size parameter that indicates how many characters should be read. Below is the source for the read function on Posix systems (Linux, etc):
The easy way to use this module is to simply set the timeout to None, and read size to 1. This will return any data received immediately. But, this setup is very inefficient when transferring large amounts of data due to the Python processing overhead.
To meet our goal of reading multi-byte blocks of data at a time, we need to pass the read function a size greater than 1. However, if timeout is set to None, the read will block until size bytes have been read, which does not meet the goal of returning any data read in a timely fashion. The solution then is to:
- set the read size high enough to get good performance
- set the timeout low enough so that any data received is returned in a reasonable timeframe, but yet the application spends most of its time blocked if there is no data.
As an example, a size of 1000 and a timeout of 1 second seems to perform well. When used this way, the pyserial module performs well and returns all data read quickly.
